API methods and Python functions to extract data from Econdb.
Start with importing the data module from pandas_datareader
```python
import pandas_datareader.data as web
```
From there retrieving data from Econdb is done by calling `DataReader`, with 'econdb' as the `data_source` keyword argument.
```python
df = web.DataReader(query, data_source='econdb')
```
The format of the first query parameter will depend on whether the data to be retrieved is a single series,
or a multi-series dataset.
## Series
Various economic time-series are accessible from many different points on the website,
like the regional country comparison table on the [home page](https://www.econdb.com/home)
or within detailed [country profiles](https://www.econdb.com/main-indicators?country=US&dateEnd=2022-07-01&dateStart=2020-07-01&freq=Q&tab=country-profile).
A series page will have a url of format /series/<ticker>, e.g. www.econdb.com/series/CPIUS, any such series can be requested by using the `ticker` part (in this example 'CPIUS')
in the following manner
```
df = web.DataReader(
"ticker=CPIUS&token=",
"econdb",
start=pd.Timestamp("2020-01-01"),
end=pd.Timestamp("2022-07-01"),
)
```
The api key is available to registered users and can be accessed
at this [account page](https://www.econdb.com/account/keys/).
## Datasets
For each source, data is partitioned into several datasets. Datasets contain tickers that share particular features like topic, frequency or survey.
We will use [Eurostat's National accounts dataset](https://www.econdb.com/dataset/NAMQ_10_GDP/gdp-and-main-components-output-expenditure-and-income/) as an example.
Within the page there are several filters available, like 'Geopolitical Entity (reporting)', 'Unit of Measure', etc.
that help narrow down the selection to a specific subset of series within the specified time-frame.
With appropriate filters in place, the 'Export' dropdown button presents a number of available formats for exporting selected data.
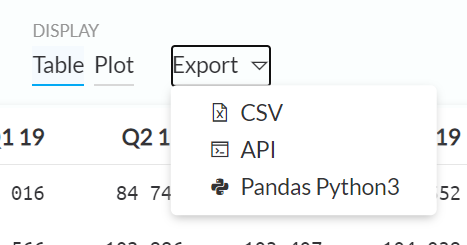
Among them, 'Pandas Python3' will generate the appropriate code snippet for retrieving the filtered subset
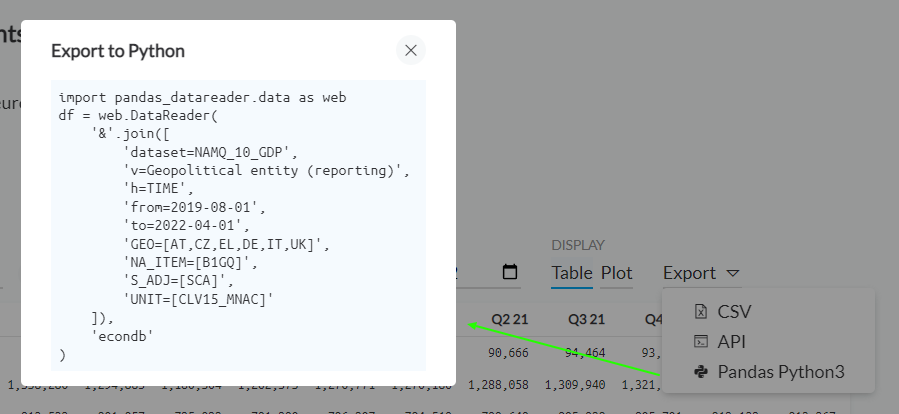
Time-frame limits can be overridden by passing the `start` and `end` keyword arguments to the DataReader.
```
df = web.DataReader(
'&'.join([
'dataset=RBI_BULLETIN',
'v=TIME',
'h=Indicator',
'from=2022-01-01',
'to=2022-07-01'
]),
'econdb',
start='2020-01-01',
end='2022-01-01'
)
```
Many different kinds of date representations are accepted here (e.g., 'JAN-01-2010', '1/1/10', 'Jan, 1, 1980').
Any argument format accepted by
[pandas.to_datetime](https://pandas.pydata.org/pandas-docs/stable/reference/api/pandas.to_datetime.html)
is accepted in the `DataReader` as well.
## Installation
Install the latest release version via pip
```python
pip install pandas-datareader
```